Revealed: Oct 2, 2024
When beginning to use a brand new CSS characteristic it is necessary to grasp its affect on the efficiency of your web sites, whether or not constructive or destructive. With @property
now in Baseline this submit explores its efficiency affect, and issues you are able to do to assist stop destructive affect.
Benchmarking the efficiency of CSS with PerfTestRunner
To benchmark the efficiency of CSS we constructed the “CSS Selector Benchmark” test suite. It’s powered by Chromium’s PerfTestRunner
and benchmarks the efficiency affect of CSS. This PerfTestRunner
is what Blink–Chromium’s underlying rendering engine–makes use of for its inner efficiency assessments.
The runner features a measureRunsPerSecond
technique which is used for the assessments. The upper the variety of runs per second, the higher. A primary measureRunsPerSecond
-benchmark with this library seems to be like this:
const testResults = PerfTestRunner.measureRunsPerSecond({
"Check Description",
iterationCount: 5,
bootstrap: operate() {
// Code to execute earlier than all iterations run
// For instance, you possibly can inject a method sheet right here
},
setup: operate() {
// Code to execute earlier than a single iteration
},
run: operate() {
// The precise check that will get run and measured.
// A typical check adjusts one thing on the web page inflicting a method or format invalidation
},
tearDown: operate() {
// Code to execute after a single iteration has completed
// For instance, undo DOM changes made inside run()
},
finished: operate() {
// Code to be run in any case iterations have completed.
// For instance, take away the fashion sheets that have been injected within the bootstrap section
},
});
Every possibility for measureRunsPerSecond
is described by feedback within the code block, with the run
operate being the core half that will get measured.
CSS Selector benchmarks require a DOM tree
As a result of the efficiency of CSS selectors can also be depending on the scale of the DOM, these benchmarks want a decently sized DOM tree. As a substitute of manually creating this DOM tree, this tree will get generated.
For instance, the next makeTree
operate is a part of the @property
benchmarks. It constructs a tree of 1000 components, every factor with some youngsters nested inside.
const $container = doc.querySelector('#container');
operate makeTree(parentEl, numSiblings) {
for (var i = 0; i <= numSiblings; i++) {
$container.appendChild(
createElement('div', {
className: `tagDiv wrap${i}`,
innerHTML: ``,
})
);
}
}
makeTree($container, 1000);
As a result of the CSS selector benchmarks do not modify the DOM tree, this tree era will get executed solely as soon as, earlier than any of the benchmarks run.
Operating a benchmark
To run a benchmark that’s a part of the test suite you should first begin an online server:
npm run begin
As soon as began you possibly can go to the benchmark at its printed URL and execute window.startTest()
manually.
To run these benchmarks in isolation—with none extensions or different elements intervening— Puppeteer is triggered from the CLI to load and execute the handed in benchmark.
For these @property
benchmarks particularly somewhat than visiting the relevant page at its URL http://localhost:3000/benchmarks/at-rule/at-property.html
invoke the next instructions on the CLI:
npm run benchmark at-rule/at-property
This masses the web page by Puppeteer, routinely calls window.startTest()
, and studies again the outcomes.
Benchmarking the efficiency of CSS properties
To benchmark the efficiency of a CSS property, you benchmark how briskly it may deal with a style invalidation and the following recalculate fashion process the browser must do.
Fashion invalidation is the method of marking which components want their fashion recalculated in response to a change within the DOM. The best doable method is to invalidate all the pieces in response to each change.
When doing so, there’s a distinction to make between CSS properties that inherit and CSS properties that do not inherit.
- When a CSS property that inherits adjustments on a focused factor, the kinds of doubtless all components within the subtree beneath the focused factor additionally want to alter.
- When a CSS property that does not inherit adjustments on a focused factor, solely the kinds for that particular person factor get invalidated.
As a result of it would not be truthful to match properties that do inherit in opposition to properties that do not, there are two units of benchmarks to run:
- A set of benchmarks with properties that inherit.
- A set of benchmarks with properties that do not inherit.
It is necessary to rigorously select which properties to benchmark. Whereas some properties (resembling accent-color
) solely invalidate kinds, there are lots of properties (resembling writing-mode
) that additionally invalidate different issues resembling format or paint. You need the properties that solely invalidate kinds.
To find out this, look issues up in Blink’s list of CSS properties. Every property has a discipline invalidate
that lists what will get invalidated.
Moreover, it is also necessary to choose a property that isn’t marked as unbiased
from that record, as benchmarking such a property would skew the outcomes. Unbiased properties haven’t any side-effects on different properties or flags. When solely unbiased properties have modified, Blink makes use of a quick code-path that clones the fashion of the descendant and updates the brand new values in that cloned copy. This method is quicker than doing a full recalculation.
Benchmarking the efficiency of CSS properties that inherit
The primary set of benchmarks focuses on CSS properties that inherit. There are three forms of properties that inherit to check and evaluate in opposition to one another:
- A daily property that inherits:
accent-color
. - An unregistered {custom} property:
--unregistered
. - A {custom} property that’s registered with
inherits: true
:--registered
.
Unregistered {custom} properties are added to this record as a result of these inherit by default.
As talked about earlier than, the property that inherits was rigorously chosen in order that it is one which solely invalidates kinds and one that isn’t marked as unbiased
.
As for registered {custom} properties, solely these with the inherits
descriptor set to true are examined on this run. The inherits
descriptor determines whether or not the property inherits down onto youngsters or not. It does not matter whether or not this property is registered by CSS @property
or JavaScript CSS.registerProperty
, because the registration itself is just not a part of the benchmark.
The benchmarks
As already talked about, the page that contains the benchmarks begins off by setting up a DOM tree in order that the web page has a sufficiently big set of nodes to see any affect of the adjustments.
Every benchmark adjustments the worth of a property after which it triggers a method invalidation. The benchmark mainly measures how lengthy the subsequent recalculation of the web page takes to re-evaluate all these invalidated kinds.
After a single benchmark is finished, any injected kinds get reset in order that the subsequent benchmark can start.
For instance, the benchmark measuring the efficiency of adjusting the fashion of --registered
seems to be like this:
let i = 0;
PerfTestRunner.measureRunsPerSecond({
description,
iterationCount: 5,
bootstrap: () => {
setCSS(`@property --registered {
syntax: "";
initial-value: 0;
inherits: true;
}`);
},
setup: operate() {
// NO-OP
},
run: operate() {
doc.documentElement.fashion.setProperty('--registered', i);
window.getComputedStyle(doc.documentElement).getPropertyValue('--registered'); // Pressure fashion recalculation
i = (i == 0) ? 1 : 0;
},
teardown: () => {
doc.documentElement.fashion.removeProperty('--registered');
},
finished: (outcomes) => {
resetCSS();
resolve(outcomes);
},
});
The benchmarks testing the opposite forms of properties work in the identical method however have an empty bootstrap
as a result of there is no such thing as a property to register.
The outcomes
Operating these benchmarks with 20 iterations on a 2021 MacBook Professional (Apple M1 Professional) with 16GB of RAM offers the next averages:
- Common property that inherits (
accent-color
): 163 runs per second (= 6.13ms per run) - Unregistered {custom} property (
--unregistered
): 256 runs per second (= 3.90ms per run) - Registered {custom} property with
inherits: true
(--registered
): 252 runs per second (= 3.96ms per run)
On a number of runs, the benchmarks yield comparable outcomes.
The outcomes present that registering a {custom} property comes at a really tiny price when in comparison with not registering the {custom} property. Registered {custom} properties that inherit run at 98% of the pace of unregistered {custom} properties. In absolute numbers, registering the {custom} property provides a 0.06ms overhead.
Benchmarking the efficiency of CSS properties that do not inherit
The subsequent properties to benchmark are people who do not inherit. Right here there are solely two forms of properties that may be benchmarked:
- A daily property that does not inherit:
z-index
. - A registered {custom} property with
inherits: false
:--registered-no-inherit
.
Customized properties that aren’t registered can’t be a part of this benchmark as a result of these properties at all times inherit.
The benchmarks
The benchmarks are similar to the earlier situations. For the check with --registered-no-inherit
, the next property registration is injected within the bootstrap
section of the benchmark:
@property --registered-no-inherit {
syntax: "";
initial-value: 0;
inherits: false;
}
The outcomes
Operating these benchmarks with 20 iterations on a 2021 MacBook Professional (Apple M1 Professional) with 16GB of RAM offers the next averages:
- Common property that does not inherit: 290,269 runs per second (= 3.44µs per run)
- Registered Customized Property that does not inherit: 214,110 runs per second (= 4.67µs per run)
The check was repeated over a number of runs and these have been the standard outcomes.
The factor that stands out right here is that properties that do not inherit carry out a lot a lot sooner than properties that do inherit. Whereas this was to be anticipated for normal properties, this additionally holds true for {custom} properties.
- For normal properties the variety of runs went up from 163 runs per second to greater than 290 thousand runs per second, a 1780% improve in efficiency!
- For {custom} properties the variety of runs went up from 252 runs per second to greater than 214 thousand runs per second, a 848% improve in efficiency!
The important thing takeaway behind that is that utilizing inherits: false
when registering a {custom} property has a significant affect. In the event you can register your {custom} property with inherits: false
, you positively ought to.
Bonus benchmark: a number of {custom} property registrations
One other attention-grabbing factor to benchmark is the affect of getting loads of {custom} property registrations. To take action, rerun the check with --registered-no-inherit
with it doing 25,000 different {custom} property registrations upfront. These {custom} properties are used on :root
.
These registrations are finished within the setup
step of the benchmark:
setup: () => {
const propertyRegistrations = [];
const declarations = [];
for (let i = 0; i < 25000; i++) {
propertyRegistrations.push(`@property --custom-${i} { syntax: ""; initial-value: 0; inherits: true; }`);
declarations.push(`--custom-${i}: ${Math.random()}`);
}
setCSS(`${propertyRegistrations.be a part of("n")}
:root {
${declarations.be a part of("n")}
}`);
},
The runs per second for this benchmark is similar to the consequence for “Registered Customized Property that doesn’t inherit” (214,110 runs per second versus 213,158 runs per second), however that is not the attention-grabbing half to take a look at. In any case, it is to be anticipated that altering one {custom} property is just not affected by registrations from different properties.
The attention-grabbing a part of this check is to measure the affect of the registrations themselves. Turning to DevTools, you possibly can see that 25,000 {custom} property registrations have an preliminary fashion recalculation price of a bit over 30ms
. As soon as that is finished, the presence of those registrations has no additional impact on issues.
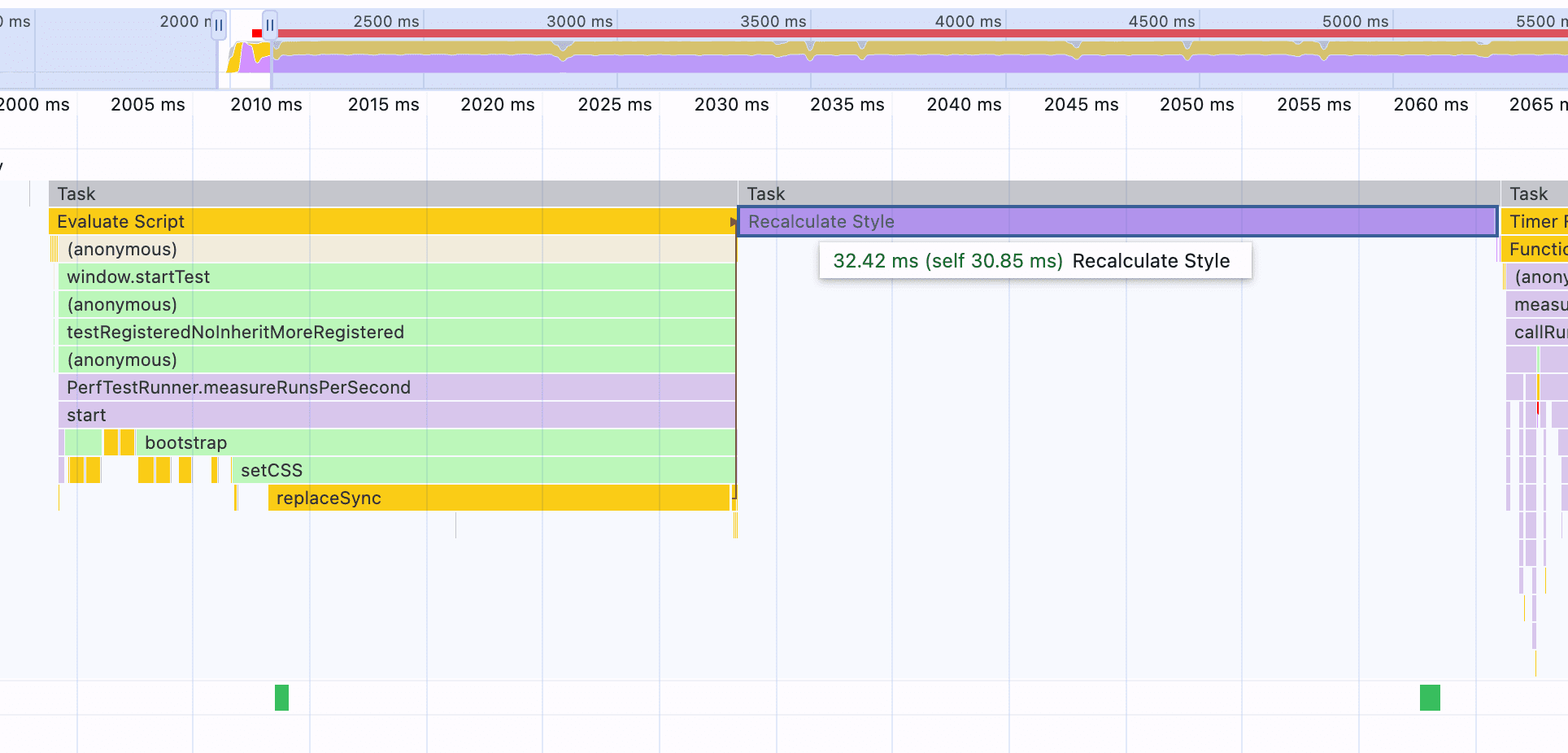
32.42ms
Conclusion and takeaways
In abstract there are three takeaways from all this:
-
Registering a {custom} property with
@property
comes at a slight efficiency price. This price is usually negligible as a result of by registering {custom} properties you unlock their full potential which isn’t doable to realize with out doing that. -
Utilizing
inherits: false
when registering a {custom} property has a significant affect. With it you stop the property from inheriting. When the property’s worth adjustments it subsequently solely impacts the kinds of the matched factor as an alternative of the complete subtree. -
Having few versus a number of
@property
registrations doesn’t affect fashion recalculation. There’s solely a really small upfront price when doing the registrations however as soon as that is finished you are good.